Laravel Filament 3 Table Tabs と User Panel Access、Authorization、Badge 学習メモ
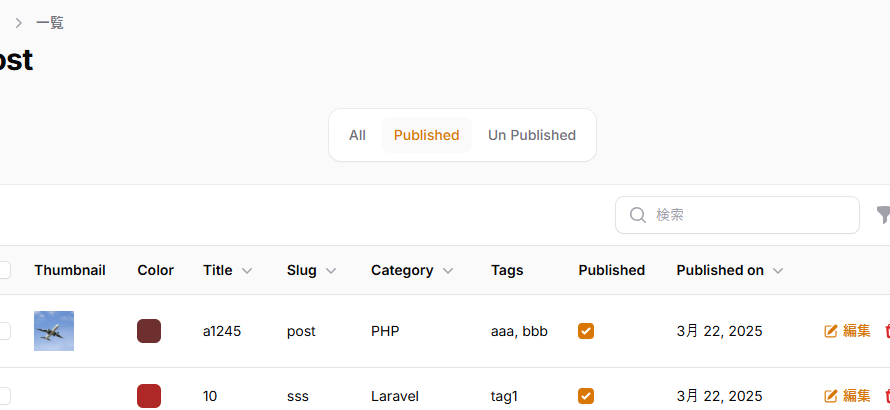
Table Tabs と User Panel Access、Badge
Table Tabs
App\Filament\Resources\PostResource\Pages\ListPosts.php(変更)
<?php
namespace App\Filament\Resources\PostResource\Pages;
use App\Filament\Resources\PostResource;
use Filament\Actions;
use Filament\Resources\Pages\ListRecords;
// use Filament\Resources\Pages\ListRecords\Tab;
use Filament\Resources\Components\Tab;
use Illuminate\Database\Eloquent\Builder;
class ListPosts extends ListRecords
{
protected static string $resource = PostResource::class;
protected function getHeaderActions(): array
{
return [
Actions\CreateAction::make(),
];
}
public function getTabs(): array
{
return [
'All' => Tab::make(),
'Published' => Tab::make()->modifyQueryUsing( function (Builder $query) {
$query->where('published', true);
}),
'Un Published' => Tab::make()->modifyQueryUsing( function (Builder $query) {
$query->where('published', false);
}),
];
}
}
User Panel Access
User.php(変更)
<?php
namespace App\Models;
// use Illuminate\Contracts\Auth\MustVerifyEmail;
use Filament\Models\Contracts\FilamentUser;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
use Filament\Panel;
// class User extends Authenticatable
class User extends Authenticatable implements FilamentUser
{
/** @use HasFactory<\Database\Factories\UserFactory> */
use HasFactory, Notifiable;
public function canAccessPanel(Panel $panel): bool
{
return $this->name === 'admin';
// return true;
}
/**
* The attributes that are mass assignable.
*
* @var list<string>
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for serialization.
*
* @var list<string>
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* Get the attributes that should be cast.
*
* @return array<string, string>
*/
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
public function posts () {
return $this->belongsToMany(Post::class, 'post_user')->withPivot(['order'])->withTimestamps();
}
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
}
アクセス不可(false)
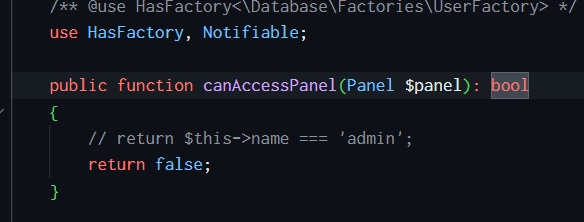
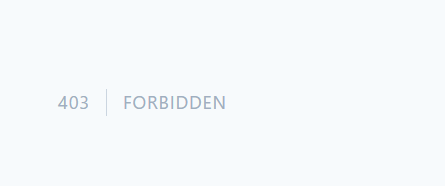
アクセス可(true)
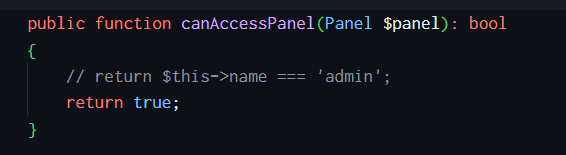
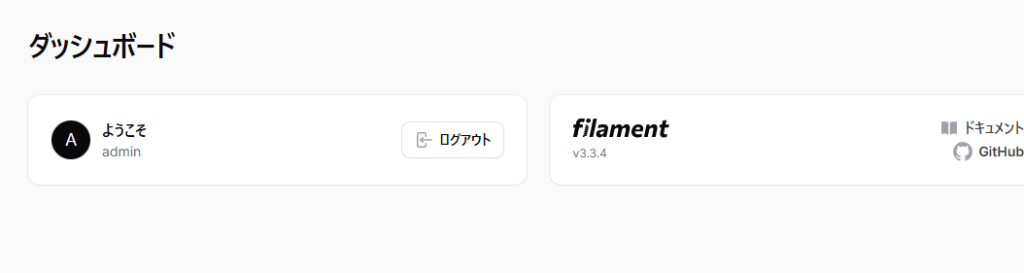
Authorization
sail artisan make:policy CategoryPolicy --model=Category

app/Policies/CategoryPolicy.php
<?php
namespace App\Policies;
use App\Models\Category;
use App\Models\User;
use Illuminate\Auth\Access\Response;
class CategoryPolicy
{
/**
* Determine whether the user can view any models.
*/
public function viewAny(User $user): bool
{
return $user->isAdmin() || $user->isEditor();
}
/**
* Determine whether the user can view the model.
*/
public function view(User $user, Category $category): bool
{
return $user->isAdmin() || $user->isEditor();
}
/**
* Determine whether the user can create models.
*/
public function create(User $user): bool
{
return $user->isAdmin() || $user->isEditor();
}
/**
* Determine whether the user can update the model.
*/
public function update(User $user, Category $category): bool
{
return $user->isAdmin() || $user->isEditor();
}
/**
* Determine whether the user can delete the model.
*/
public function delete(User $user, Category $category): bool
{
return $user->isAdmin();
}
public function deleteAny(User $user): bool
{
return $user->isAdmin();
}
/**
* Determine whether the user can restore the model.
*/
public function restore(User $user, Category $category): bool
{
return $user->isAdmin();
}
/**
* Determine whether the user can permanently delete the model.
*/
public function forceDelete(User $user, Category $category): bool
{
return $user->isAdmin();
}
}
editor@test.co.jp/パスワードでログイン
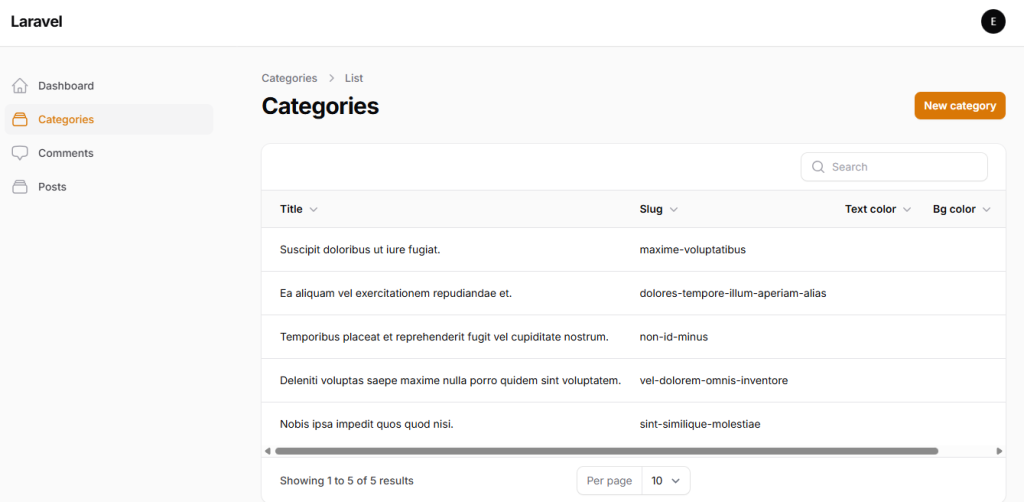
admin@test.co.jp/パスワードでログイン
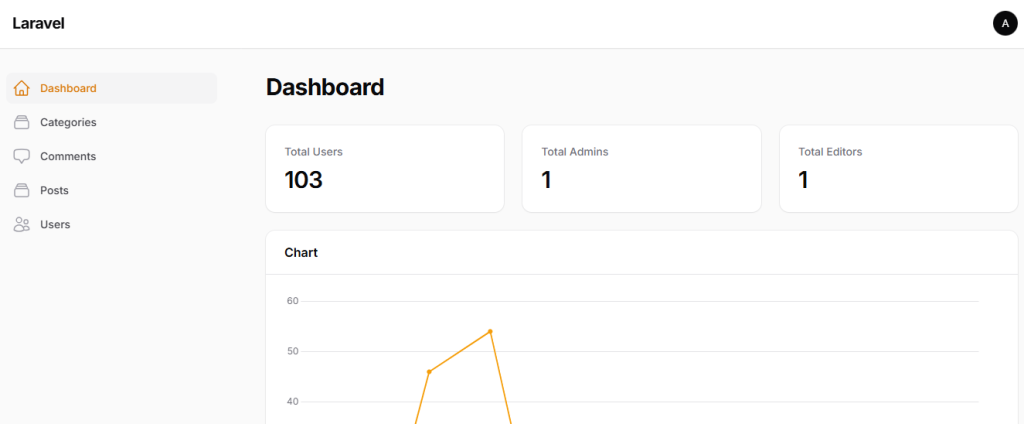
その他のソース
CategoryResource.php(変更)
<?php
namespace App\Filament\Resources;
use App\Filament\Resources\CategoryResource\Pages;
use App\Filament\Resources\CategoryResource\RelationManagers;
use App\Models\Category;
use Filament\Forms;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Form;
use Filament\Resources\Resource;
use Filament\Tables;
use Filament\Tables\Columns\TextColumn;
use Filament\Tables\Table;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
use Illuminate\Support\Str;
class CategoryResource extends Resource
{
protected static ?string $model = Category::class;
protected static ?string $navigationIcon = 'heroicon-o-rectangle-stack';
// protected static bool $shouldSkipAuthorization = true;
public static function form(Form $form): Form
{
return $form
->schema([
TextInput::make('title')
->live()
->required()->minLength(1)->maxLength(150)
->afterStateUpdated(function (string $operation, $state, Forms\Set $set) {
if ($operation === 'edit') {
return;
}
$set('slug', Str::slug($state));
}),
TextInput::make('slug')->required()->minLength(1)->unique(ignoreRecord: true)->maxLength(150),
TextInput::make('text_color')->nullable(),
TextInput::make('bg_color')->nullable(),
]);
}
public static function table(Table $table): Table
{
return $table
->columns([
TextColumn::make('title')->sortable()->searchable(),
TextColumn::make('slug')->sortable()->searchable(),
TextColumn::make('text_color')->sortable()->searchable(),
TextColumn::make('bg_color')->sortable()->searchable(),
])
->filters([
//
])
->actions([
Tables\Actions\EditAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
]),
]);
}
public static function getRelations(): array
{
return [
//
];
}
public static function getPages(): array
{
return [
'index' => Pages\ListCategories::route('/'),
'create' => Pages\CreateCategory::route('/create'),
'edit' => Pages\EditCategory::route('/{record}/edit'),
];
}
}
User.php(参考に)
<?php
namespace App\Models;
// use Illuminate\Contracts\Auth\MustVerifyEmail;
use Filament\Models\Contracts\FilamentUser;
use Filament\Panel;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Fortify\TwoFactorAuthenticatable;
use Laravel\Jetstream\HasProfilePhoto;
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable implements FilamentUser
{
use HasApiTokens;
use HasFactory;
use HasProfilePhoto;
use Notifiable;
use TwoFactorAuthenticatable;
const ROLE_ADMIN = 'ADMIN';
const ROLE_EDITOR = 'EDITOR';
const ROLE_USER = 'USER';
const ROLE_DEFAULT = self::ROLE_USER;
const ROLES = [
self::ROLE_ADMIN => 'Admin',
self::ROLE_EDITOR => 'Editor',
self::ROLE_USER => 'User',
];
public function canAccessPanel(Panel $panel): bool
{
return $this->can('view-admin', User::class);
}
public function isAdmin(){
return $this->role === self::ROLE_ADMIN;
}
public function isEditor(){
return $this->role === self::ROLE_EDITOR;
}
/**
* The attributes that are mass assignable.
*
* @var array<int, string>
*/
protected $fillable = [
'name',
'email',
'password',
'role'
];
/**
* The attributes that should be hidden for serialization.
*
* @var array<int, string>
*/
protected $hidden = [
'password',
'remember_token',
'two_factor_recovery_codes',
'two_factor_secret',
];
/**
* The attributes that should be cast.
*
* @var array<string, string>
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
/**
* The accessors to append to the model's array form.
*
* @var array<int, string>
*/
protected $appends = [
'profile_photo_url',
];
public function likes()
{
return $this->belongsToMany(Post::class, 'post_like')->withTimestamps();
}
public function hasLiked(Post $post)
{
return $this->likes()->where('post_id', $post->id)->exists();
}
public function comments()
{
return $this->hasMany(Comment::class);
}
}
Badge
UserResource.php(変更)
<?php
namespace App\Filament\Resources;
use App\Filament\Resources\UserResource\Pages;
use App\Filament\Resources\UserResource\RelationManagers;
use App\Models\User;
use Filament\Forms;
use Filament\Forms\Form;
use Filament\Resources\Resource;
use Filament\Tables;
use Filament\Tables\Table;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
class UserResource extends Resource
{
protected static ?string $model = User::class;
protected static ?string $navigationIcon = 'heroicon-o-users';
public static function form(Form $form): Form
{
return $form
->schema([
Forms\Components\TextInput::make('name')
->required()
->maxLength(255),
Forms\Components\TextInput::make('email')
->email()
->required()
->maxLength(255),
Forms\Components\Select::make('role')
->options(User::ROLES)
->required(),
]);
}
public static function table(Table $table): Table
{
return $table
->columns([
Tables\Columns\TextColumn::make('name')
->searchable(),
Tables\Columns\TextColumn::make('email')
->searchable(),
Tables\Columns\TextColumn::make('created_at')
->dateTime()
->sortable()
->toggleable(isToggledHiddenByDefault: true),
Tables\Columns\TextColumn::make('updated_at')
->dateTime()
->sortable()
->toggleable(isToggledHiddenByDefault: true),
Tables\Columns\TextColumn::make('role')
->badge()
->color(function(string $state) : string {
// return 'info'; //
// if ($state == 'ADMIN') return 'danger';
// return 'gray';
return match($state) {
'ADMIN' => 'danger', //RED
'EDITOR' => 'info', //BLUE
'USER' => 'success', //GREEN
};
})
->sortable()
->searchable(),
])
->filters([
//
])
->actions([
Tables\Actions\EditAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
]),
]);
}
public static function getRelations(): array
{
return [
//
];
}
public static function getPages(): array
{
return [
'index' => Pages\ListUsers::route('/'),
'create' => Pages\CreateUser::route('/create'),
'edit' => Pages\EditUser::route('/{record}/edit'),
];
}
}
カラーは、
https://filamentphp.com/docs/3.x/support/colors
use Filament\Support\Colors\Color;
use Filament\Support\Facades\FilamentColor;
FilamentColor::register([
'danger' => Color::Red,
'gray' => Color::Zinc,
'info' => Color::Blue,
'primary' => Color::Amber,
'success' => Color::Green,
'warning' => Color::Amber,
]);