Laravel フレームワーク初期導入の手順の覚書 便利なlaravel-adminを導入してみる
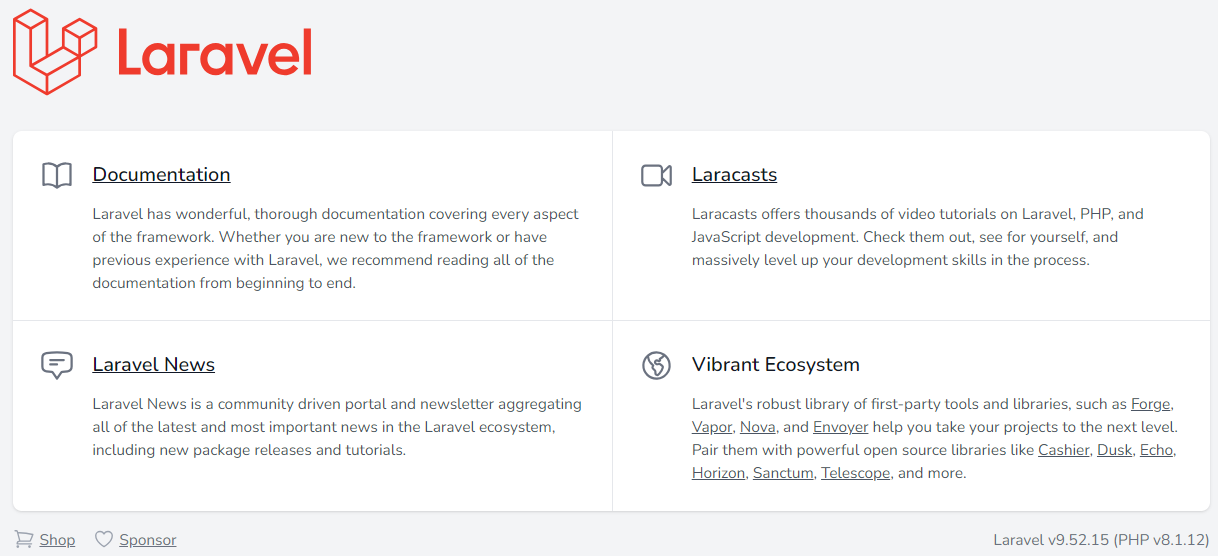
ここでは一般的に laravel を初期導入する際に必要な事を覚書。
参考サイト
laravel 初期導入手順の覚書
ここでは、composer や php、xamppはインストール済みの環境が整っている物とする。
laravel プロジェクトの新規作成。バージョン指定付き。
composer create-project "laravel/laravel=9.*" sales --prefer-dist

mysql myadmin で新規にデータベース作る
新規にデータベースを作成する。照合順序=utf8mb4_general_ci。

laravel プロジェクトDB情報(.env)の設定
.env の DB_*** の箇所を設定する。

laravel-admin インストール
Laravelの管理画面用パッケージ「laravel-admin」をインストールする。
まず、プロジェクトフォルダへ移動する。
cd sales
composer require encore/laravel-admin

次にasset、configファイルを移動させる。
php artisan vendor:publish --provider="Encore\Admin\AdminServiceProvider"
最後に下記コマンドを実行することでインストールが完了。
php artisan admin:install
インストール後のデータベース。各テーブルが用意されました。

WEBサーバーをいずれかの方法で起動します。
php artisan serve
または、xampp の apache を起動。
その後、下記のURLにアクセスします。
http://localhost/sales/public/admin/
※ちなみに、laravel デフォルトのTOPは、
http://localhost/sales/public/
初期の管理者として、admin/admin が登録済み。adminでログインしてみる。


コマンドで管理者を作成する方法。初期管理者のadmin以外を
php artisan admin:create
> taro@test.com
> taro@test.com
> taro@test.com


ログインしてみる。taro@test.com

ログインてきました。

サンプルアプリ(Blog)を作ってみる
blogサンプルアプリを追加して、laravel-adminのメニュー追加する。
まずは、
テーブルを作成(マイグレーション)する
php artisan make:migration create_blogs_table --create=blogs

生成された、database>migrations>マイグレーションファイル を編集する。カラムを追加する。

<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('blogs', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('text');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('blogs');
}
};
マイグレーション実行する
php artisan migrate

データベースに反映されている事を確認。

Modelからアプリ作成する
larave-adminはadmin:makeコマンドを使用してアプリを作成する。
Models > 配下に下記のモデルを作成する
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Blog extends Model
{
use HasFactory;
protected $fillable = [
'title',
'text'
];
}
Blogモデルのアプリを作成します。
php artisan admin:make BlogController --model=App\Models\Blog

app/Admin/routes.php に追記します。
<?php
use Illuminate\Routing\Router;
Admin::routes();
Route::group([
'prefix' => config('admin.route.prefix'),
'namespace' => config('admin.route.namespace'),
'middleware' => config('admin.route.middleware'),
'as' => config('admin.route.prefix') . '.',
], function (Router $router) {
$router->get('/', 'HomeController@index')->name('home');
$router->resource('users', UserController::class);
$router->resource('blogs', BlogController::class);
});
作成されたコントローラー(BlogController)です。
<?php
namespace App\Admin\Controllers;
use App\Models\Blog;
use Encore\Admin\Controllers\AdminController;
use Encore\Admin\Form;
use Encore\Admin\Grid;
use Encore\Admin\Show;
class BlogController extends AdminController
{
/**
* Title for current resource.
*
* @var string
*/
protected $title = 'Blog';
/**
* Make a grid builder.
*
* @return Grid
*/
protected function grid()
{
$grid = new Grid(new Blog());
$grid->column('id', __('Id'));
$grid->column('title', __('Title'));
$grid->column('text', __('Text'));
$grid->column('created_at', __('Created at'));
$grid->column('updated_at', __('Updated at'));
return $grid;
}
/**
* Make a show builder.
*
* @param mixed $id
* @return Show
*/
protected function detail($id)
{
$show = new Show(Blog::findOrFail($id));
$show->field('id', __('Id'));
$show->field('title', __('Title'));
$show->field('text', __('Text'));
$show->field('created_at', __('Created at'));
$show->field('updated_at', __('Updated at'));
return $show;
}
/**
* Make a form builder.
*
* @return Form
*/
protected function form()
{
$form = new Form(new Blog());
$form->text('title', __('Title'));
$form->textarea('text', __('Text'));
return $form;
}
}
メニューに追加します。Admin>Menu 。




こんな感じです。

その他、パラメータ値の変更、覚書
さくらレンタルサーバーで動かそうとすると認証画面でログインできない。laravel-adminのhttps化を有効にする必要がある
/config/admin.php の false → trueへ
'https' => env('ADMIN_HTTPS', true),
Adminアカウントのユーザー設定画面で『Disk [admin] not configured, please add a disk config in config/filesystems.php
.』エラーが出た‥
/config/filesystems.php に admin情報を追記
'disks' => [
'local' => [
'driver' => 'local',
'root' => storage_path('app'),
'throw' => false,
],
'public' => [
'driver' => 'local',
'root' => storage_path('app/public'),
'url' => env('APP_URL').'/storage',
'visibility' => 'public',
'throw' => false,
],
's3' => [
'driver' => 's3',
'key' => env('AWS_ACCESS_KEY_ID'),
'secret' => env('AWS_SECRET_ACCESS_KEY'),
'region' => env('AWS_DEFAULT_REGION'),
'bucket' => env('AWS_BUCKET'),
'url' => env('AWS_URL'),
'endpoint' => env('AWS_ENDPOINT'),
'use_path_style_endpoint' => env('AWS_USE_PATH_STYLE_ENDPOINT', false),
'throw' => false,
],
'admin' => [
'driver' =>'local',
'root' => public_path('uploads'),
'visibility' =>'public',
'url' => env('APP_URL').'/uploads',
],
その他、laravel 初期設定
ここで気づいたのですが、laravelの基本的、または推奨する初期設定となります。
タイムゾーン、言語設定
config/app.php
// アプリケーションデフォルトのタイムゾーンを設定できます。
// PHPの日付および日時関数を使用する際にこの設定を参照します。
'timezone' => 'Asia/Tokyo',
// 翻訳サービスプロバイダーが使用するデフォルトのロケールを設定します。
'locale' => 'ja',
// フォールバックロケールは、指定したロケールが使用できない場合に使用するロケールを決定します。
'fallback_locale' => 'ja',
// FakerPHPライブラリがデータを生成する際に使用されます。
'faker_locale' => 'ja_JP',
-
前の記事
Windows Excel VBA + Selenium WEBスクレイピング chrome edge 2023.08.17
-
次の記事
laravel-admin 検索画面について 条件追加 カスタマイズ grid/filter 2023.08.24